반응형
1. ListView에 Custom layout에 필요한 데이터 모델 생성
package application.model;
public class CategoryModel {
private String title; // 카테고리 타이틀
public CategoryModel(String title) {
this.title = title;
}
}
2. ListVIew 에 표시될 Custom Layout Cell 생성
- FXMLLoader으로 Custom Layout Cell을 불러온다. 그리고 setGraphic으로 부모 Pane 을 넘겨주어야한다.
import application.model.CategoryModel;
import javafx.fxml.FXML;
import javafx.fxml.FXMLLoader;
import javafx.scene.control.ListCell;
import javafx.scene.layout.AnchorPane;
/**
* 카테고리 Cell
* @author 임성진
*
*/
public class CategoryCell extends ListCell<CategoryModel>{
private FXMLLoader fxLoader;
@FXML
private AnchorPane apPane;
@Override
protected void updateItem(CategoryModel model, boolean empty) {
// TODO Auto-generated method stub
super.updateItem(model, empty);
if (!empty && model != null) {
if (this.fxLoader == null) {
this.fxLoader = new FXMLLoader(getClass().getResource("../view/category_item.fxml"));
this.fxLoader.setController(this);
try {
this.fxLoader.load();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
setGraphic(apPane);
}
}
}
3. Controller에서 테스트 데이터를 생성 후, setCallFactory 으로 Cell Callback을 등록한다.
package application.controller;
import java.net.URL;
import java.util.ResourceBundle;
import application.cell.CategoryCell;
import application.model.CategoryModel;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.fxml.FXML;
import javafx.fxml.Initializable;
import javafx.scene.control.ListView;
public class MainController implements Initializable{
@FXML
private ListView<CategoryModel> lvCategory;
// 카테고리 리스트
private ObservableList<CategoryModel> categoryList;
public MainController() {
// 카테고리에 표시할 리스트 생성
this.categoryList = FXCollections.observableArrayList();
// test 데이터 생성
for (int i=0; i<100; i++)
this.categoryList.add(new CategoryModel("TEST"));
}
@Override
public void initialize(URL arg0, ResourceBundle arg1) {
// 카테고리 아이템 등록
lvCategory.setItems(this.categoryList);
lvCategory.setCellFactory(categoryList -> new CategoryCell());
}
}
4. custom layout cell이될 layout을 만든다.
- category_item.fxml
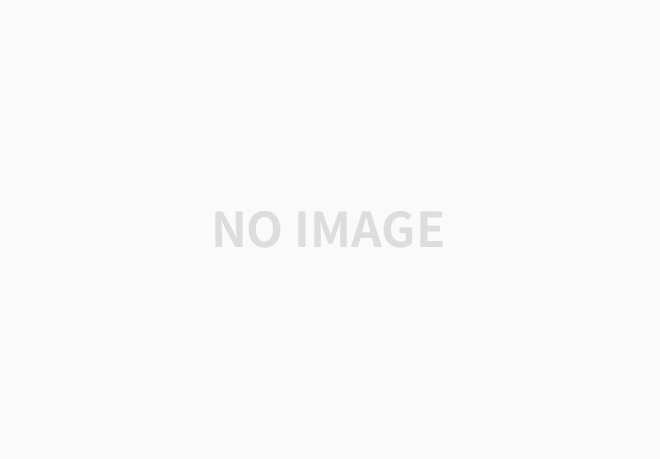
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.geometry.Insets?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.image.Image?>
<?import javafx.scene.image.ImageView?>
<?import javafx.scene.layout.AnchorPane?>
<?import javafx.scene.layout.HBox?>
<?import javafx.scene.text.Font?>
<AnchorPane fx:id="apPane" xmlns:fx="http://javafx.com/fxml/1" xmlns="http://javafx.com/javafx/15.0.1">
<children>
<HBox prefHeight="30.0" prefWidth="224.0">
<children>
<ImageView fitHeight="25.0" fitWidth="25.0" pickOnBounds="true" preserveRatio="true">
<image>
<Image url="@../img/ic_search.png" />
</image>
<HBox.margin>
<Insets left="10.0" />
</HBox.margin>
</ImageView>
<Label prefHeight="25.0" text="Export setup">
<HBox.margin>
<Insets left="5.0" />
</HBox.margin>
<font>
<Font size="15.0" />
</font>
</Label>
</children>
<padding>
<Insets top="3.0" />
</padding>
</HBox>
</children>
</AnchorPane>
5. 결과
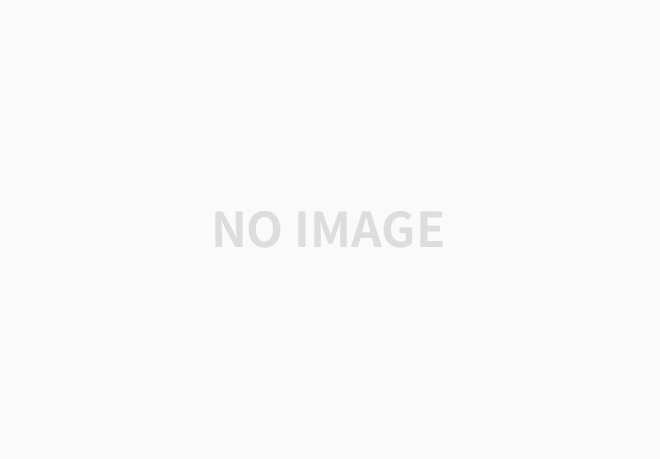
반응형
'Programming > javaFX' 카테고리의 다른 글
[javaFX] Getting Started Modular with Gradle Tutorial (Eclipse) (0) | 2021.03.02 |
---|---|
[javaFX] CellSpanTableView for javaFX 11 (0) | 2021.02.26 |
[javaFX] CSS Reference Guid (0) | 2021.02.24 |
[javaFX] JAXB (Java Architecture for XML Binding) - java 11 (0) | 2021.02.20 |
[javaFX] javaFX 11 eclipse tutorial (0) | 2021.02.18 |